Excel VBAを使用して、連番を持つファイル名の番号をリセットする方法について説明します。業務でのファイル管理や整理に役立つこの処理は、特定のディレクトリ内のファイルを一元管理したい際などに非常に便利です。この記事では、基本的な手法から応用例まで、具体的なコードとその詳細な解説を通して、この技術の理解を深めていきます。
Excel VBAの基本
Excel VBA(Visual Basic for Applications)は、Microsoft Excelに組み込まれたプログラミング言語です。これを用いると、単純作業の自動化だけでなく、高度なデータ分析やレポート作成も可能になります。
そもそも、どこにVBAコードを書いて、どう実行すれば良いのか分からない場合は、以下の記事をご参照ください。
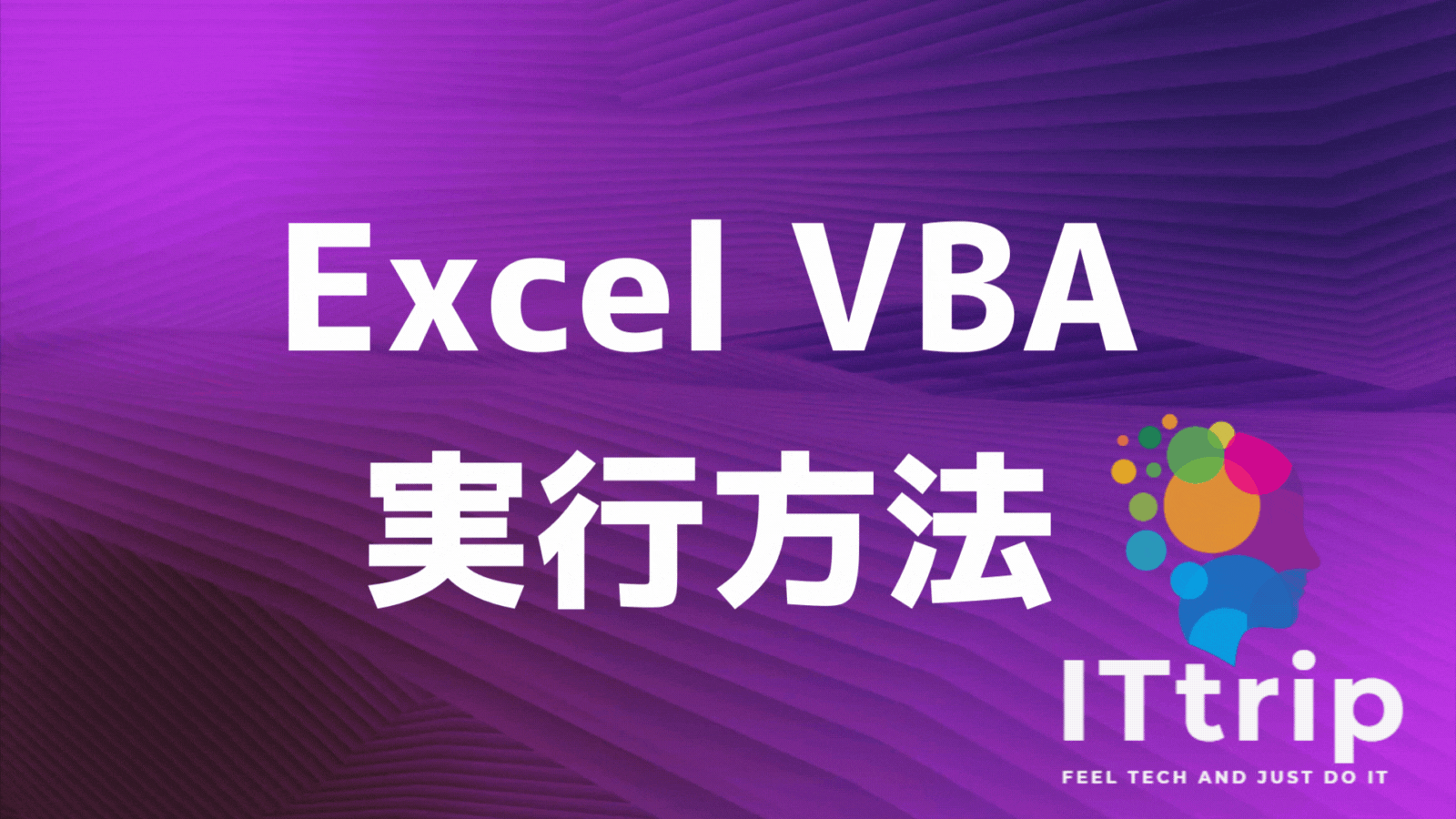
基本的な処理方法
VBAを使用して、特定のフォルダ内の連番を持つファイル名をリセットするための基本的なコードは以下の通りです。
Sub ResetFileNumbering()
Dim folderPath As String
Dim fileName As String
Dim counter As Integer
' フォルダパスの指定
folderPath = "C:\example\"
If Right(folderPath, 1) <> "\" Then folderPath = folderPath & "\"
fileName = Dir(folderPath & "*.xlsx")
counter = 1
Do While fileName <> ""
Name folderPath & fileName As folderPath & "file" & counter & ".xlsx"
fileName = Dir
counter = counter + 1
Loop
End Sub
このコードでは、`C:\example\`のパスにあるExcelファイル(.xlsx)のファイル名を、`file1.xlsx`、`file2.xlsx`といった形にリセットしています。`Dir`関数を用いることで、指定したフォルダ内のファイル名を取得し、`Name`ステートメントでファイル名を変更しています。
応用例1: 拡張子を考慮したリセット
もし、異なる拡張子のファイルが混在しているディレクトリで、それぞれの拡張子ごとに連番をリセットしたい場合は、以下のように処理を行います。
Sub ResetFileNumberingByExtension()
Dim folderPath As String
Dim fileName As String
Dim counter As Integer
Dim ext As String
folderPath = "C:\example\"
If Right(folderPath, 1) <> "\" Then folderPath = folderPath & "\"
ext = ".xlsx"
fileName = Dir(folderPath & "*" & ext)
counter = 1
Do While fileName <> ""
Name folderPath & fileName As folderPath & "file" & counter & ext
fileName = Dir
counter = counter + 1
Loop
End Sub
応用例2: 指定したプレフィックスを考慮する
特定のプレフィックスを持つファイルだけをリセットしたい場合には、以下のコードを使用します。
Sub ResetFileNumberingByPrefix()
Dim folderPath As String
Dim fileName As String
Dim counter As Integer
Dim prefix As String
folderPath = "C:\example\"
If Right(folderPath, 1) <> "\" Then folderPath = folderPath & "\"
prefix = "report_"
fileName = Dir(folderPath & prefix & "*.xlsx")
counter = 1
Do While fileName <> ""
Name folderPath & fileName As folderPath & prefix & counter & ".xlsx"
fileName = Dir
counter = counter + 1
Loop
End Sub
応用例3: 連番のスタート番号や増加幅を変更する
連番の開始番号や増加幅を変更したい場合には、以下のようなコードを使用します。
Sub ResetFileNumberingByIncrement()
Dim folderPath As String
Dim fileName As String
Dim counter As Integer
Dim incrementValue As Integer
folderPath = "C:\example\"
If Right(folderPath, 1) <> "\" Then folderPath = folderPath & "\"
fileName = Dir(folderPath & "*.xlsx")
counter = 10 ' 連番の開始番号
incrementValue = 5 ' 増加幅
Do While fileName <> ""
Name folderPath & fileName As folderPath & "file" & counter & ".xlsx"
fileName = Dir
counter = counter + incrementValue
Loop
End Sub
まとめ
Excel VBAを利用して、連番を持つファイル名の番号をリセットする処理は、業務効率化のための強力なツールとなります。基本的な方法から応用まで、さまざまなケースでのリセット方法を学ぶことで、日々の業務の中でのファイル管理が一層効率的になるでしょう。
VBAも良いけどパワークエリも良い
VBAの解説をしてきましたが、VBAは正直煩雑でメンテナンス性が悪いです。最近はモダンExcelと呼ばれるパワークエリやパワーピボットへのシフトが進んできています。本サイトでもパワークエリの特集をしており、サンプルデータを含む全11回の学習コンテンツでパワークエリを習得することができます。
クリックするとパワークエリの全11講座が表示されます。
-
【初心者向け】パワークエリ入門:ETLツールを使ってエクセルデータを簡単に整形・統合しよう!(1/11)
-
【実践ガイド】パワークエリでデータ収集:Excel、CSV、PDF、Webデータを簡単に取り込む方法をマスターしよう!(2/11)
-
【総力特集】パワークエリで列操作をマスター:選択、変更、移動、削除、結合、分割の詳細解説&実践テクニック!(3/11)
-
【徹底解説】パワークエリで行操作をマスター!フィルター・保持・削除テクニックと練習用エクセルで実践学習(4/11)
-
パワークエリでデータクレンジング: 文字列結合、0埋め、テキスト関数をマスター(5/11)
-
パワークエリで四捨五入、切り捨て、切り上げをマスターする方法(6/11)
-
パワークエリで効率的なデータグループ化を実現する方法(7/11)
-
パワークエリで時間と日付の計算をマスター!便利な関数を使って効率アップ(8/11)
-
パワークエリで条件別集計をマスターする方法(9/11)
-
Excelパワークエリでクロス集計表とデータベース形式を瞬時に変換する方法(10/11)
-
Excelパワークエリ入門: 効率的なデータ整理をマスターしよう!(11/11)
パワーピボットの記事はありません。興味がある場合は、書籍で学んでみてください
コメント